[Python:파이썬:기초] 18. 클래스 다중 상속, 추상 클래스 - Class Multiple Inheritance, Abstract Class Examples
안녕하세요 JollyTree입니다 (•̀ᴗ•́)و
다중상속(Multiple Inheritance)
다중 상속은 여러개의 클래스로부터 상속을 받는 것을 말합니다. 다음과 같이 콤마(,)를 이용하여 2개 이상의 베이스 클래스 이름을 입력할 수 있습니다.
class 베이스클래스1: 코드 class 베이스클래스2: 코드 class 파생클래스(베이스클래스1, 베이스클래스2): 코드 |
🔗 클래스 다중 상속 예제(Example):
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
|
#베이스 클래스(Base Class)
class Car:
def __init__(self):
print("Init. Car Class")
#파생 클래스 - Car 클래스를 상속받음
class Sedan(Car):
def __init__(self):
super().__init__()
print("Init. Sedan Class")
def sendanInfo(self):
print("편해요")
#파생 클래스 - Car 클래스를 상속받음
class Suv(Car):
def __init__(self):
super().__init__()
print("Init. Suv Class")
def suvInfo(self):
print("넓어요")
#다중상속 클래스 : Sedan, Suv를 상속받음
class Hybrid(Sedan, Suv):
def __init__(self, maxSpeed):
self.maxSpeed = maxSpeed
super().__init__()
print("Init. Hybrid Class")
def getSpeed(self):
return self.maxSpeed
hybrid = Hybrid(300) #객체 생성
print("Hybrid max speed = ", hybrid.getSpeed())
hybrid.sendanInfo() #Sedan 클래스의 메소드 호출
hybrid.suvInfo() #Suv 클래스의 메소드 호출
#메소드 탐색 순서 출력
print(Suv.mro())
#메소드 탐색 순서 출력:베이스클래스 순서(Sedan->Suv순)
print(Hybrid.mro())
|
cs |
🔗 실행결과(Output):
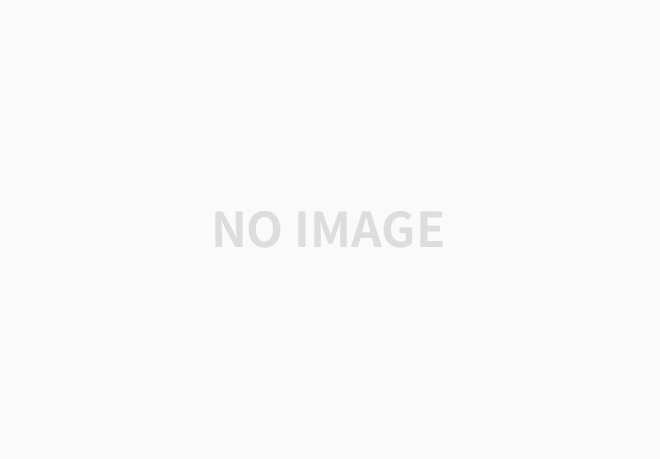
추상 클래스(Abstract Class)
추상 클래스는 객체지향 언어의 특성을 이해해야 합니다. 일반적으로 규모가 큰 프로그램을 설계할 때 베이스 클래스를 설계하는 단계에서 파생 클래스를 구체화할 수없는 경우 상속 후 메소드 정의를 강제화 하는 용도로 사용됩니다. 추상 클래스의 기본 구조는 다음과 같습니다.
from abc import * class 추상클래스(metaclass=ABCMeta): @abstractmethod def 메소드이름1(self): pass @abstractmethod def 메소드이름2(self): pass class 파생클래스(추상클래스): def 메소드이름1(self): 코드 def 메소드이름2(self): 코드 |
추상 클래스는 독립적이면서 공통된 기능의 파생 클래스들의 구현이 필요할 때 사용되며 추상 클래스 자체 메소드 안의 코드는 구현하지 않습니다. 즉, 파생 클래스를 만들 때 반드시 메소드가 구현되어야 하며 구현되지 않으면 에러가 발생합니다.
TypeError: Can't instantiate abstract class Engineer with abstract methods need_wheels
또한, 용어에서도 알 수 있듯이 추상 클래스는 실제 구체화된 클래스가 아니므로 객체를 생성할 수 없습니다. 만약 객체 생성을 시도하면 다음과 같은 에러가 발생합니다.
TypeError: Can't instantiate abstract class Car with abstract methods need_handle, need_wheels
🔗 클래스 추상 클래스 예제(Example):
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
|
from abc import *
class Car(metaclass = ABCMeta):
@abstractmethod
def need_wheels(self): #추상 메소드
pass
#메소드 구현
@abstractmethod
def need_handle(self): #추상 메소드
pass
#Car 클래스를 상속받음
class Sedan(Car):
#메소드 구현
def need_wheels(self, numWheels, numDoors):
self.numWheels = numWheels
self.numDoors = numDoors
print("Sedan: 분홍색 바퀴")
def need_handle(self): #메소드 구현
print("Sedan: 초록색 핸들")
#Car 클래스를 상속받음
class Suv(Car):
#메소드 구현
def need_wheels(self, numWheels, numDoors):
self.numWheels = numWheels
self.numDoors = numDoors
print("Suv: 노랑색 바퀴")
#메소드 구현
def need_handle(self):
print("Suv: 하얀색 핸들")
#carObj = Car()
mySedan = Sedan()
mySedan.need_wheels(4, 1)
mySedan.need_handle()
mySedan = Suv()
mySedan.need_wheels(4, 1)
mySedan.need_handle()
|
cs |
🔗 실행결과(Output):
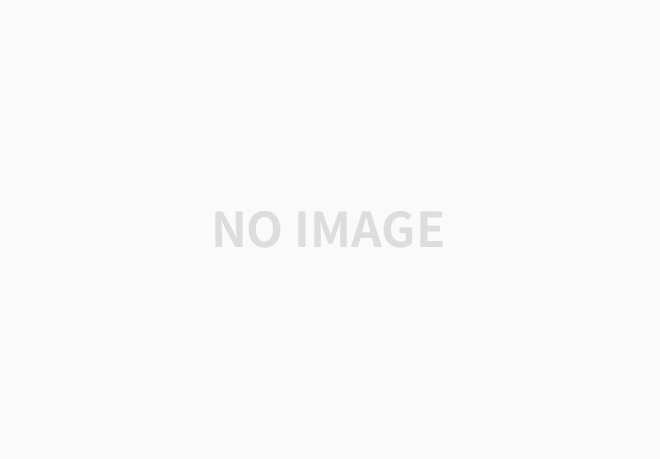
이상 JollyTree였습니다 (•̀ᴗ•́)و